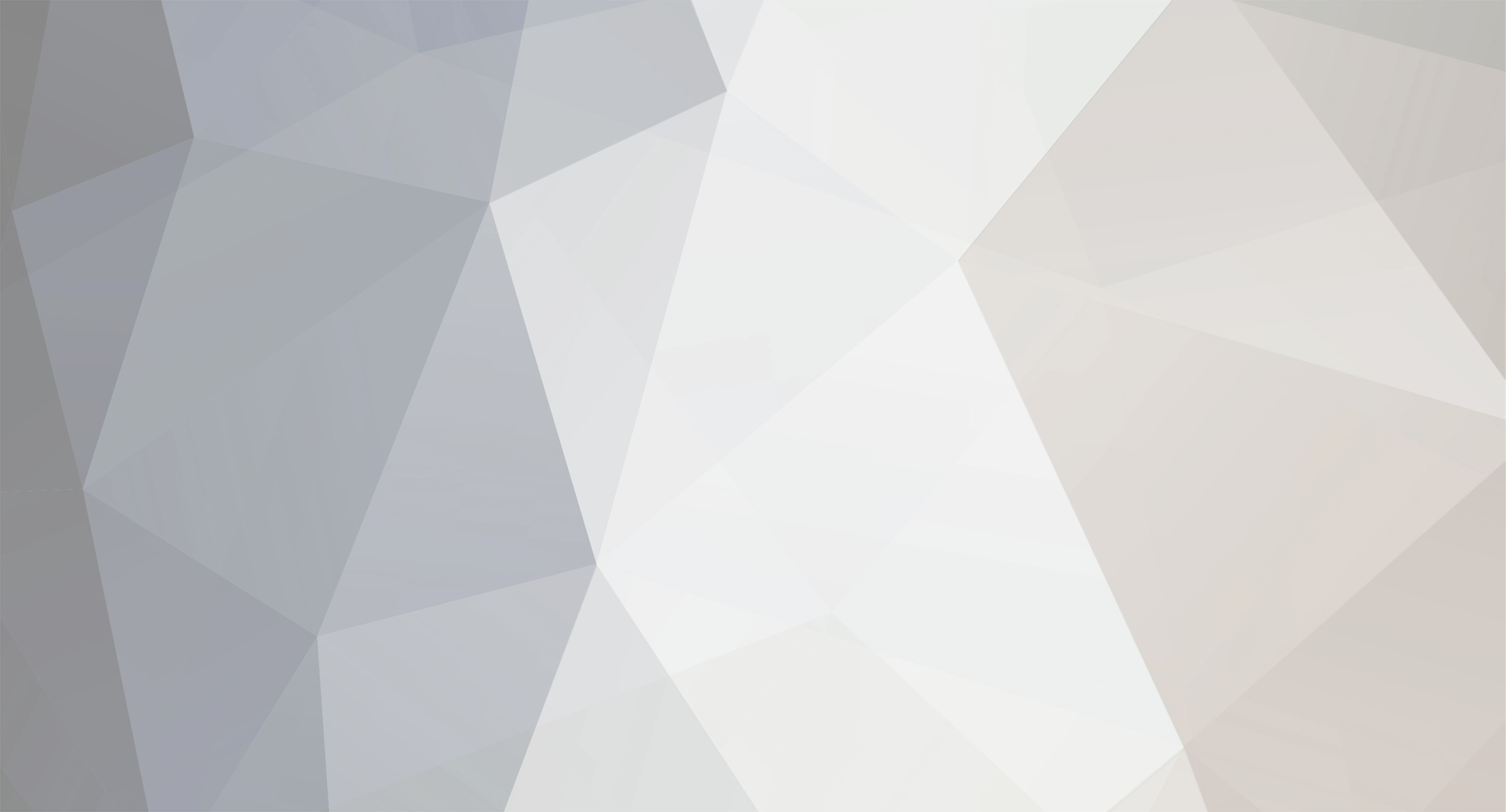
Aidenholmes9
-
Posts
9 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Posts posted by Aidenholmes9
-
-
Version 2, Now the values, price and energy requeriment change with the rarity, when unequiping the upgrade now yaw,pitch and roll should be what you have before installing the upgrade.
package.path = package.path .. ";data/scripts/systems/?.lua" package.path = package.path .. ";data/scripts/lib/?.lua" include ("basesystem") include ("utility") -- optimization so that energy requirement doesn't have to be read every frame FixedEnergyRequirement = true Unique = true function getBonuses(seed, rarity) local thrusters = Thrusters() local pitch = 0 local yaw = 0 local roll = 0 if rarity.value == RarityType.Petty then pitch = (rarity.value + 1.25) yaw = (rarity.value + 1.25) roll = (rarity.value + 1.25) end if rarity.value == RarityType.Common then pitch = (rarity.value + 0.50) yaw = (rarity.value + 0.50) roll = (rarity.value + 0.50) end if rarity.value == RarityType.Uncommon then pitch = (rarity.value - 0.25) yaw = (rarity.value - 0.25) roll = (rarity.value - 0.25) end if rarity.value == RarityType.Rare then pitch = (rarity.value - 1) yaw = (rarity.value - 1) roll = (rarity.value - 1) end if rarity.value == RarityType.Exceptional then pitch = (rarity.value - 1.75) yaw = (rarity.value - 1.75) roll = (rarity.value - 1.75) end if rarity.value == RarityType.Exotic then pitch = (rarity.value - 2.50) yaw = (rarity.value - 2.50) roll = (rarity.value - 2.50) end if rarity.value == RarityType.Legendary then pitch = (rarity.value - 3) yaw = (rarity.value - 3) roll = (rarity.value - 3) end return pitch, yaw, roll end function onInstalled(seed, rarity, permanent) if not permanent then return end local pitch, yaw, roll = getBonuses(seed, rarity) local thrusters = Thrusters() thrusters.basePitch = pitch + thrusters.currentPitch thrusters.baseRoll = roll + thrusters.currentRoll thrusters.baseYaw = yaw + thrusters.currentYaw thrusters.fixedStats = 1 end function onUninstalled(seed, rarity, permanent) local pitch, yaw, roll = getBonuses(seed, rarity) local thrusters = Thrusters() thrusters.basePitch = thrusters.currentPitch - pitch thrusters.baseRoll = thrusters.currentRoll - roll thrusters.baseYaw = thrusters.currentYaw - yaw thrusters.fixedStats = 0 end function getName(seed, rarity) return "Gyro Array Upgrade"%_t end function getIcon(seed, rarity) return "data/textures/icons/gyroscope.png" end function getEnergy(seed, rarity, permanent) local pitch, yaw, roll = getBonuses(seed, rarity) return (pitch + yaw + roll * 1500000000) end function getPrice(seed, rarity) local pitch, yaw, roll = getBonuses(seed, rarity) return (pitch + yaw + roll * 200000) end function getTooltipLines(seed, rarity, permanent) local pitch, yaw, roll = getBonuses(seed, rarity) local texts = { {ltext = "Pitch"%_t, rtext = "${pitch1}"%_t % {pitch1 = (pitch)}, icon = "data/textures/icons/gyroscope.png", boosted = permanent}, {ltext = "Yaw"%_t, rtext = "${yaw1}"%_t % {yaw1 = (pitch)}, icon = "data/textures/icons/gyroscope.png", boosted = permanent}, {ltext = "Roll"%_t, rtext = "${roll1}"%_t % {roll1 = (pitch)}, icon = "data/textures/icons/gyroscope.png", boosted = permanent} } if not permanent then return {}, texts else return texts, texts end end function getDescriptionLines(seed, rarity, permanent) return { {ltext = "", boosted = permanent}, {ltext = "Fixed Pitch Yaw and Roll."%_t, lcolor = ColorRGB(1, 0.5, 0.5)} } end
Here the upgrade generator:
add("data/scripts/systems/gyroarrayupgrade.lua", 1)
Here the link: https://drive.google.com/open?id=1WLoN-PUgGS5L795M1LSN0r1ICT2rgm-P
(This is the steam version of the mod...)
(I have this uploaded to steam but only friend can see it because i never spent a cent on the steam)
If you upload this to workshop give me some little credits please =P
-
Great, keep it up and thanks for your work ;D
-
My Native Language is Spanish, i have the engine covered with 0.5 blocks and i can see the glow from the front... Bloom is unchecked also i can still see the exausth of the engine when i boost even if it is covered... i have attached some images one with glow other accelerating so the front glow cannot be seen and other two that show how the engine exausht still can be seen even with the block covered. All is covered on hull...
-
So is there a way to remove the engine "glow" when you look at your ship from the front? Also is possible to make the engine "Exit effect" only work if the block itself is expoced and not when blocked?
The request would be: remove engine effects...
Sorry for my bad english.
-
So i should make a script that add the bonus?
Yes
Also where the upgrades "initialize" and "restore"?System upgrades include "basesystem.lua" - it has restore
So the "onInstalled function" is the"restore" and not "initialize" thanks for the help! i should need the "secure" and "restore" functions right? if you can lead me to how to change "onInstalled" to the so called "entity reload" would save me weeks even if you point to a game file that do it, but for now you made my day thanks!
-
You should look at the "Borg Conquest and Assimilation" mod on the steam workshop it doenst have custom gates but you can open a destructible wormhole to your home sector that have a "plan" so it could be a start to jumpgates with custom "plans", look at the thebeacon.lua script in entity folder that where it starts...
-
StatBonuses changes are tied to the script. The moment that script unloads or if entity is reloaded, they disappear and need to be re-applied.
Look at how system upgrades apply bonuses - they do it initially in the "initialize" and after that in "restore".
So i should make a script that add the bonus? or can i make it on the item? Also where the upgrades "initialize" and "restore"?
Here a simple upgrade that adds velocity and acceleration like and absolute value...
package.path = package.path .. ";data/scripts/systems/?.lua" package.path = package.path .. ";data/scripts/lib/?.lua" include ("basesystem") include ("utility") -- optimization so that energy requirement doesn't have to be read every frame FixedEnergyRequirement = true Unique = true function getBonuses(seed, rarity) local velocity = 200 local acceleration = 25 local energy = 2 return velocity, acceleration, energy end function onInstalled(seed, rarity, permanent) if not permanent then return end local velocity, acceleration, energy = getBonuses(seed, rarity) addAbsoluteBias(StatsBonuses.Velocity, velocity) addAbsoluteBias(StatsBonuses.Acceleration, acceleration) addBaseMultiplier(StatsBonuses.GeneratedEnergy, energy) end function onUninstalled(seed, rarity, permanent) end function getName(seed, rarity) return "Antimatter Engine"%_t end function getIcon(seed, rarity) return "data/textures/icons/turbine.png" end function getPrice(seed, rarity) return 10000 end function getTooltipLines(seed, rarity, permanent) local velocity, acceleration, energy = getBonuses(seed, rarity) local texts = { {ltext = "Velocity"%_t, rtext = "?"%_t, icon = "data/textures/icons/speedometer.png", boosted = permanent}, {ltext = "Acceleration"%_t, rtext = "?"%_t, icon = "data/textures/icons/acceleration.png", boosted = permanent}, {ltext = "Generated Energy"%_t, rtext = "?"%_t, icon = "data/textures/icons/electric.png", boosted = permanent} } if not permanent then return {}, texts else return texts, texts end end function getDescriptionLines(seed, rarity, permanent) return { {ltext = "This antimatter engine generates energy."%_t, lcolor = ColorRGB(1, 0.5, 0.5)}, {ltext = "", boosted = permanent}, {ltext = "This system gives fixed acceleration /* continues with 'and velocity ignoring weight.' */"%_t, rtext = "", icon = ""}, {ltext = "and velocity ignoring weight. /* continued from 'This system gives fixed acceleration' */"%_t, rtext = "", icon = ""} } end
I suppose that the "initialize" is the "oninstalled function"? but where is the "restore"? I dont really know how to programe i can barely read those scripts and modify them like the upgrade or the first item that was a merchant caller... if you can take the time to explain or write an example great and thanks in advance and sorry again for my english :P
-
So i did an item that spawn a player ship and during generation adds some scripts to the ship, works well until i try to set a statbonus, here the script
package.path = package.path .. ";data/scripts/lib/?.lua" package.path = package.path .. ";data/scripts/?.lua" include("stringutility") local ShipGenerator = include("shipgenerator") local NamePool = include ("namepool") function create(item, rarity, allyIndex) rarity = Rarity(RarityType.Legendary) item.stackable = false item.depleteOnUse = true item.name = "Nomad Beacon"%_t item.price = 1000000000 item.icon = "data/textures/icons/ship.png" item.rarity = rarity item:setValue("subtype", "ReinforcementsTransmitter") item:setValue("factionIndex", allyIndex) local tooltip = Tooltip() tooltip.icon = item.icon local title = "Nomad Beacon"%_t local headLineSize = 25 local headLineFontSize = 15 local line = TooltipLine(headLineSize, headLineFontSize) line.ctext = title line.ccolor = item.rarity.color tooltip:addLine(line) -- empty line tooltip:addLine(TooltipLine(14, 14)) local line = TooltipLine(20, 14) line.ltext = "Player Ship"%_t line.rtext = "${faction:"..allyIndex.."}" line.icon = "data/textures/icons/flying-flag.png" line.iconColor = ColorRGB(0.8, 0.8, 0.8) tooltip:addLine(line) tooltip:addLine(TooltipLine(14, 14)) local line = TooltipLine(20, 14) line.ltext = "Nomad Type"%_t line.rtext = "Prototype" line.icon = "data/textures/icons/ship.png" line.iconColor = ColorRGB(0.8, 0.8, 0.8) tooltip:addLine(line) tooltip:addLine(TooltipLine(14, 14)) local line = TooltipLine(20, 14) line.ltext = "Turrets Factory"%_t line.rtext = "Yes" line.rcolor = ColorRGB(0.3, 1, 0.3) line.icon = "data/textures/icons/turret.png" line.iconColor = ColorRGB(0.8, 0.8, 0.8) tooltip:addLine(line) local line = TooltipLine(20, 14) line.ltext = "Fighter Factory"%_t line.rtext = "Yes" line.rcolor = ColorRGB(0.3, 1, 0.3) line.icon = "data/textures/icons/fighter.png" line.iconColor = ColorRGB(0.8, 0.8, 0.8) tooltip:addLine(line) local line = TooltipLine(20, 14) line.ltext = "Upgrades Factory"%_t line.rtext = "No" line.rcolor = ColorRGB(1, 0.3, 0.3) line.icon = "data/textures/icons/circuitry.png" line.iconColor = ColorRGB(0.8, 0.8, 0.8) tooltip:addLine(line) local line = TooltipLine(20, 14) line.ltext = "Torpedoes Factory"%_t line.rtext = "No" line.rcolor = ColorRGB(1, 0.3, 0.3) line.icon = "data/textures/icons/missile-pod.png" line.iconColor = ColorRGB(0.8, 0.8, 0.8) tooltip:addLine(line) local line = TooltipLine(20, 14) line.ltext = "Utilities Factory"%_t line.rtext = "No" line.rcolor = ColorRGB(1, 0.3, 0.3) line.icon = "data/textures/icons/satellite.png" line.iconColor = ColorRGB(0.8, 0.8, 0.8) tooltip:addLine(line) local line = TooltipLine(20, 14) line.ltext = "Research Facility"%_t line.rtext = "Yes" line.rcolor = ColorRGB(0.3, 1, 0.3) line.icon = "data/textures/icons/cog.png" line.iconColor = ColorRGB(0.8, 0.8, 0.8) tooltip:addLine(line) local line = TooltipLine(20, 14) line.ltext = "Refinery Facility"%_t line.rtext = "Yes" line.rcolor = ColorRGB(0.3, 1, 0.3) line.icon = "data/textures/icons/zinc.png" line.iconColor = ColorRGB(0.8, 0.8, 0.8) tooltip:addLine(line) -- empty line tooltip:addLine(TooltipLine(14, 14)) local line = TooltipLine(20, 15) line.ltext = "Depleted on Use"%_t line.lcolor = ColorRGB(1.0, 1.0, 0.3) tooltip:addLine(line) -- empty line tooltip:addLine(TooltipLine(14, 14)) local line = TooltipLine(20, 14) line.ltext = "Can be activated by the player"%_t tooltip:addLine(line) local line = TooltipLine(20, 14) line.ltext = "Calls in the Nomad"%_t tooltip:addLine(line) local line = TooltipLine(20, 14) line.ltext = "Can be used anywhere"%_t tooltip:addLine(line) item:setTooltip(tooltip) return item end function activate(item) local faction = Galaxy():getControllingFaction(Sector():getCoordinates()) local sender = NamedFormat("${faction} Headquarters"%_T, {faction = faction.baseName}) local player = Player() local playerFaction = player.craftFaction local craft = player.craft -- Create the Nomad local pos = random():getDirection() * 1500 local matrix = MatrixLookUpPosition(normalize(-pos), vec3(0, 1, 0), pos) local ship = ShipGenerator.createShip(player, matrix) ship:invokeFunction("icon.lua", "set", nil) ship:removeScript("icon.lua") ship.title = "Nomad"%_T ship:addScriptOnce("data/scripts/entity/merchants/fighterfactory.lua") ship:addScriptOnce("data/scripts/entity/merchants/turretfactory.lua") ship:addScriptOnce("data/scripts/entity/merchants/researchstation.lua") ship:addScriptOnce("data/scripts/entity/merchants/refinery.lua") ship:addAbsoluteBias(StatsBonuses.HyperspaceReach, 300) -- HERE THE PROBLEM ship:addCrew(1, CrewMan(CrewProfessionType.Captain)) NamePool.setShipName(ship) Sector():broadcastChatMessage(ship, 0, "Reporting in..."%_t, ship.title, ship.name) return true end
the problem is that the "ship:addAbsoluteBias(StatsBonuses.HyperspaceReach, 300)" works but when i close the "server (Singleplayer)" and then connect again that ship doenst have the hyper space bonus anymore, is possible to make that bonus permanent/persistent ?
Thanks and sorry for my english...
{Help} ship:addAbsoluteBias persistence?
in Mods
Posted
Here the script if somebody is interested... Works well and the stats persist i dont know if it efficient but for my personal use works...