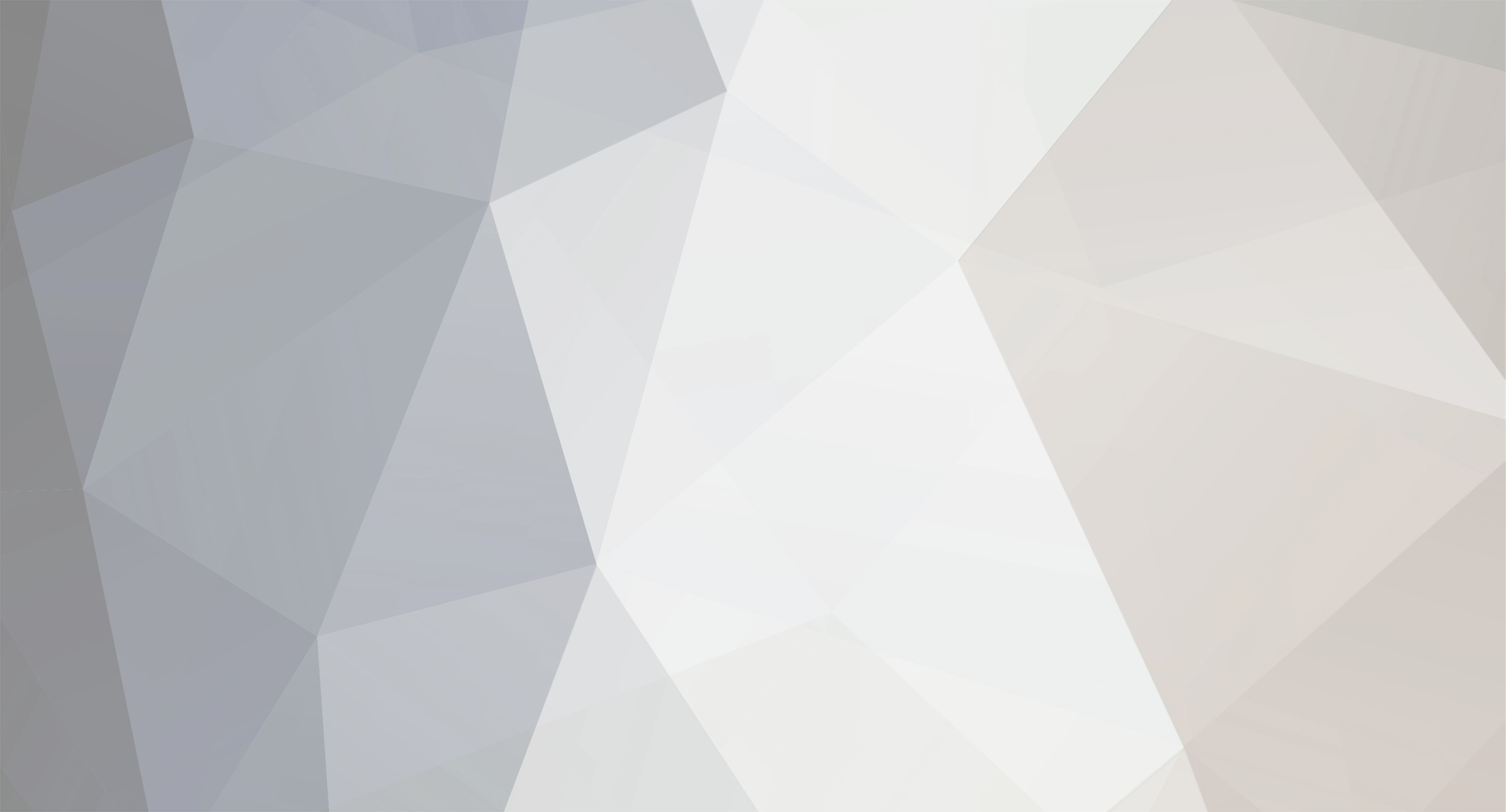
Gordo
-
Posts
5 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Posts posted by Gordo
-
-
I'm attempting to make lua file system work with Avorion's lua. Now, by default require("module") can load lua modules.
Using the following lua:
local lfs = require("lfs")
I compiled lua file system using Mingw-w64 4.9.2 using the following commands:
gcc -c -Wall -fpic lfs.c -IC:/lua/include gcc -shared -o lfs.dll lfs.o -LC:/lua/ -llua52
Which produced the lfs.dll file. Which is usable in normal lua52 and I've tested that, it loads fine.
I double checked what version of lua you're using in your engine and it reported lua52.
So then I did a simple test and used require("lfs") the module is found and the server crashes.
it then spits out this error
2017-07-08 03-29-02| Attempting to load luafilesystem 2017-07-08 03-29-02| Error: EXCEPTION_ACCESS_VIOLATION 2017-07-08 03-29-02| [1] lua_close 1688275260 2017-07-08 03-29-02| [2] lua_setglobal 1688216929 2017-07-08 03-29-02| [3] luaopen_lfs 1820600884 2017-07-08 03-29-02| [4] ?? 8428541 2017-07-08 03-29-02| [5] ?? 8429975 2017-07-08 03-29-02| [6] ?? 8406099 2017-07-08 03-29-02| [7] ?? 8453615 2017-07-08 03-29-02| [8] ?? 8428541 2017-07-08 03-29-02| [9] ?? 8511810 2017-07-08 03-29-02| [10] ?? 8429987 2017-07-08 03-29-02| [11] ?? 8406099 2017-07-08 03-29-02| [12] ?? 8453615 2017-07-08 03-29-02| [13] ?? 8428541 2017-07-08 03-29-02| [14] ?? 8511810 2017-07-08 03-29-02| [15] ?? 8429987 2017-07-08 03-29-02| [16] ?? 8425454 2017-07-08 03-29-02| [17] ?? 8430935 2017-07-08 03-29-02| [18] ?? 8406280 2017-07-08 03-29-02| [19] ?? 7578548 2017-07-08 03-29-02| [20] ?? 7579960 2017-07-08 03-29-02| [21] ?? 4383927 2017-07-08 03-29-02| [22] ?? 4237801 2017-07-08 03-29-02| [23] ?? 4931768 2017-07-08 03-29-02| [24] ?? 6440048 2017-07-08 03-29-02| [25] ?? 4860090 2017-07-08 03-29-02| [26] ?? 4864676 2017-07-08 03-29-02| [27] ?? 4223143 2017-07-08 03-29-02| [28] ?? 4242288 2017-07-08 03-29-02| [29] ?? 4244496 2017-07-08 03-29-02| [30] ?? 4209063 2017-07-08 03-29-02| [31] ?? 11199471 2017-07-08 03-29-02| [32] ?? 4199416 2017-07-08 03-29-02| [33] ?? 4199707 2017-07-08 03-29-02| [34] BaseThreadInitThunk 140716112422772
So then I changed the load library code in the DLL and commented out the default library functionality to make sure the DLL was loading, and it was, the engine stopped crashing.
LFS_EXPORT int luaopen_lfs (lua_State *L) { //dir_create_meta (L); /*lock_create_meta (L);*/ //luaL_newlib (L, fslib); //lua_pushvalue(L, -1); //lua_setglobal(L, LFS_LIBNAME); //set_info (L); return 1; }
Then i tested a basic example from the lua c api wiki and the same crash happened.
static int l_sin (lua_State *L) { double d = luaL_checknumber(L, 1); lua_pushnumber(L, sin(d)); return 1; /* number of results */ } LFS_EXPORT int luaopen_lfs (lua_State *L) { lua_pushcfunction(L, l_sin); lua_setglobal(L, "mysin"); //dir_create_meta (L); /*lock_create_meta (L);*/ //luaL_newlib (L, fslib); //lua_pushvalue(L, -1); //lua_setglobal(L, LFS_LIBNAME); //set_info (L); return 1; }
Can you please fix this as it's not fun being unable to use these modules?
LuaFileSystem is not really the main one I wanted to test but it was the smallest. (it's a 2 file library)
https://keplerproject.github.io/luafilesystem/
-
I just had a minor suggestion for your net-code optimisation, as I have had a similar issue you are facing with your game.
Currently I predict your model to be this for networking
clientside-lua <-> game <-> client-socket <-> server-socket <-> server lua
if this is so, each server tick waits for all objects and entities to finish updating.
The problem is if the objects updating takes longer than a server tick, the server starts to stutter. (you know this already; i know)
Couldn't you change the model of the game to use the lua yield statements in a co-routine to exit prematurely out of expensive AI code, so that you can send client updates of model positions and other network data, before all objects are finished updating.
This would mean that you could send entity states prematurely, this would also be chunking the update.
Thus you would potentially be saving a larger portion of time.
Also, I highly recommend using LuaJIT as it's a lot faster than standard Lua. (if you use FFI properly and wrap your userdata cleverly) updating to this alone would probably save you having to manually optimise.
The performance comparison to JIT compilation turned off.
-
I just had a minor suggestion for your net-code optimisation, as I have had a similar issue you are facing with your game.
Currently I predict your model to be this for networking
clientside-lua <-> game <-> client-socket <-> server-socket <-> server lua
if this is so, each server tick waits for all objects and entities to finish updating.
The problem is if the objects updating takes longer than a server tick, the server starts to stutter. (you know this already; i know)
Couldn't you change the model of the game to use the lua yield statements in a co-routine to exit prematurely out of expensive AI code, so that you can send client updates of model positions and other network data, before all objects are finished updating.
This would mean that you could send entity states prematurely, this would also be chunking the update.
Thus you would potentially be saving a larger portion of time.
Also, I highly recommend using LuaJIT as it's a lot faster than standard Lua. (if you use FFI properly and wrap your userdata cleverly) updating to this alone would probably save you having to manually optimise.
lua failing to load modules
in Bugs
Posted
Any updates on this, I would appreciate any feedback